How to Optimize Laravel Performance for Faster Websites
When developing web applications, Laravel is a top choice for developers due to its elegant syntax, rich features, and powerful capabilities.

When developing web applications, Laravel developers is a top choice for developers due to its elegant syntax, rich features, and powerful capabilities. However, as projects scale, performance optimization becomes essential to ensure fast load times and efficient resource usage. In this comprehensive guide, we’ll explore the best strategies to optimize Laravel performance for a faster website.
1. Use the Latest Laravel Version
Laravel is frequently updated with performance improvements, bug fixes, and security patches. Keeping your application on the latest Laravel version ensures optimal performance and access to new features. Before upgrading, always test your application in a staging environment.
2. Optimize Composer Autoload
Run the following command to generate an optimized class map and reduce lookup time:
composer dump-autoload --optimize
This command improves Laravel's ability to load classes efficiently, resulting in faster execution times.
3. Use Route Caching
For applications with many routes, caching them can improve performance. Execute:
php artisan route:cache
This compiles all routes into a single file, making route resolution significantly faster.
4. Optimize Configuration Caching
Configuration caching reduces the time required to load environment and config files. Use:
php artisan config:cache
This command compiles all configuration files into a single file, reducing load time and improving Laravel performance.
5. Optimize Database Queries
Inefficient database queries can slow down Laravel applications. Here are some best practices:
-
Use Eager Loading: Prevent N+1 query issues by loading related models efficiently.
$users = User::with('posts')->get();
-
Use Indexing: Add indexes to frequently queried columns.
-
Avoid Select * Queries: Retrieve only necessary columns using
select()
. -
Use Query Caching: Implement Laravel query caching with:
Cache::remember('users', 3600, function() { return User::all(); });
6. Use Laravel Queues for Background Processing
Offload time-consuming tasks to queues to enhance Laravel performance. Implement queues by setting up a queue driver (Redis, Amazon SQS, etc.) and dispatching jobs asynchronously:
php artisan queue:work
Queues significantly improve response times by handling tasks like email sending, notifications, and report generation in the background.
7. Implement Caching Mechanisms
Using caching can drastically reduce database queries and improve Laravel performance. Consider the following caching strategies:
-
Application Caching: Store frequently accessed data in cache:
Cache::put('key', 'value', $seconds);
-
Use Redis or Memcached: These are more efficient than file caching.
-
View Caching: Cache rendered views using:
php artisan view:cache
8. Minimize Middleware Usage
Avoid excessive middleware usage, as each middleware layer adds processing overhead. Only apply middleware where necessary and use route groups to optimize performance.
9. Optimize Session Storage
By default, Laravel stores sessions in files, which may not scale well. Switching to Redis or database-backed sessions can significantly enhance session management.
SESSION_DRIVER=redis
10. Optimize Image and Asset Loading
Large image files and unoptimized assets slow down website performance. To improve load times:
-
Use CDN for static files.
-
Compress images with tools like TinyPNG or Laravel Spatie Image Optimization package.
-
Minify CSS and JavaScript files using Laravel Mix.
11. Enable OPcache
OPcache stores compiled PHP code in memory, reducing parsing overhead. Enable it in php.ini
:
opcache.enable=1
opcache.memory_consumption=128
opcache.max_accelerated_files=4000
12. Hire Professional Laravel Developers
For businesses looking to build high-performance Laravel applications, hiring expert Laravel developers can make a significant difference. Whether you need to hire remote AWS developers or a specialized Laravel team, expert developers ensure your application is built with performance and scalability in mind.
13. Monitor and Optimize Performance with Tools
Use performance monitoring tools to continuously analyze and optimize your Laravel application:
-
Laravel Telescope: Provides deep insights into application behavior.
-
New Relic: Monitors application performance in real-time.
-
Blackfire.io: Helps profile and debug performance bottlenecks.
Conclusion
Optimizing Laravel performance is crucial for delivering a fast and scalable web application. By implementing route caching, database query optimization, caching mechanisms, queue processing, and asset optimization, you can significantly enhance speed and efficiency. If you’re looking for expert developers, Logix Built Solutions Limited provides top-tier talent to help you build optimized Laravel applications.
What's Your Reaction?




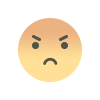

